1. Change the case of string in python in C++
Write a function that converts a given string to lower case.
Assume, given string contains uppercase alphabets only. But input can contain multiple words.
Sample Input 1 :
ABCDE
Sample Output 1 :
abcde
Sample Input 2 :
ABC DEF
Sample Output 2 :
abc def
C++ Code:
// Update in the given string itself. No need to return or print anything.
void convertStringToLowerCase(char input[]) {
// Write your code here
int len=0;
while(input[len]!='\0')
len++;
for(int i=0;i<=len;i++)
{
if(input[i] >= 'A' && input[i] <= 'Z')
input[i]=input[i] + 32;
}
}
2.Remove character from string in c++
For a given a string(str) and a character X, write a function to remove all the occurrences of X from the given string.
The input string will remain unchanged if the given character(X) doesn't exist in the input string.
Input Format:
The first line of input contains a string without any leading and trailing spaces. The second line of input contains a character(X) without any leading and trailing spaces.
Output Format:
The only line of output prints the updated string.
Note:
You are not required to print anything explicitly. It has already been taken care of.
Constraints:
0 <= N <= 10^6 Where N is the length of the input string. Time Limit: 1 second
Sample Input 1:
aabccbaa a
Sample Output 1:
bccb
Sample Input 2:
xxyyzxx y
Sample Output 2:
xxzxx
C++ Code:
// input - given string // You need to remove all occurrences of character c that are present in string input. // Change in the input itself. So no need to return or print anything. void removeAllOccurrencesOfChar(char input[], char c) { // Write your code here int len=0,j; while(input[len]!=0) len++; for(int i=0;i<len;i++) { if(input[i]!=c) { input[j]=input[i]; j++; } } input[j]='\0'; }
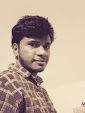
0 comments:
Post a Comment